- Welcome To Guide Dogs For The Blind | Guide Dogs For The Blind
- Further Information
- Gdb Online
- What Is The Difference Between LLDB And GDB - Pediaa.Com
- Tutorial: Debugging Embedded Devices Using GDB - Chris Simmonds, 2net Ltd
- RMS's Gdb Tutorial: How Do I Use Breakpoints?
COMMAND | DESCRIPTION |
---|---|
help | List gdb command categories |
help category | List gdb commands of category |
help command | Displays description of command |
apropos search-word | Display commands list containing search-word in their help description |
info args i args | Displays arguments of current function |
info breakpoints info break info b i b | Displays list of breakpoints |
info break breakpoint-number i b breakpoint-number | Displays description of breakpoint having breakpoint-number |
info watchpoints i watchpoints | Displays list of watchpoints |
info registers i r | Display processor's register contents |
info threads i threads | Display information about current thread |
info set i set | List set-able options |
r | Start running program until a breakpoint or end of program |
Breakpoints and Watchpoints | |
break funname break line-no break ClassName::funcName | Set a breakpoint at specified function or line number. |
break +line-offset break -line-offset | Set a breakpoint at specified number of lines forward or backward from current line of execution. |
break filename:funcname | Set a breapoint at specified funcname of given filename. |
break filename:line-no | Set a breakpoint at given line-no of specified filename. |
break *address | Set a breakpoint at specified instrunction address. |
break line-no if condition | Set a conditional breakpoint at line-no of current file. Program will suspend only when condition is true. |
break line thread thread-no | Set a breakpoint at line in thread with thread-no. |
tbreak | tbreak is similar to break but it will temporary breakpoint. i.e. Breakpoint will be deleted once it is hit. |
watch condition | Set a watchpoint for given condition. Program will suspend when condition is true. |
clear clear function clear line-number | Delete breakpoints as identified by command option. Delete all breakpoints in function Delete breakpoints at given line |
delete | Delete all breakpoints, watchpoints, or catchpoints. |
delete breakpoint-number delete range | Delete the breakpoints, watchpoints, or catchpoints specified by number orranges. |
disable breakpoint-number disable range enable breakpoint-number enable range | Enable/Disable breakpoints, watchpoints or catchpoints specified by number orranges. |
enable breakpoint-number once | Enables given breakpoint once. And disables it after it is hit. |
Program Execution | |
continue c | Continues/Resumes running the program until the next breakpoint or end of program |
continue number | Continue but ignore current breakpoint number times. |
finish | Continue until the current function is finished |
step | Runs the next line of the program |
step N | Runs the next N lines of program |
next | Like s, but it does not step into functions |
print var | Prints the current value of the variable 'var' |
set var=val | Assign 'val' value to the variable 'var' |
backtrace | Prints a stack trace |
q | Quit from gdb |
If you experience low-level problems such as crashes or deadlocks(e.g. when tinkering with parts of CPython which are written in C),it can be convenient to use a low-level debugger such as gdb inorder to diagnose and fix the issue. By default, however, gdb (or anyof its front-ends) doesn’t know about high-level information specific to theCPython interpreter, such as which Python function is currently executing,or what type or value has a given Python object represented by a standardPyObject*
pointer. We hereafter present two ways to overcome thislimitation.
Gdb is in the gnu package on CEC machines. If you don't have this package loaded then type pkgadd gnu at a shell prompt. If you can run g, then you will be able to run gdb.
gdb 7 and later¶
Sep 28, 2018 Miscellaneous gdb commands. L command: Use gdb command l or list to print the source code in the debug mode. Use l line-number to view a specific line number (or) l function to view a specific function. Bt: backtrack – Print backtrace of all stack frames, or innermost COUNT frames. Help – View help for a particular gdb topic — help TOPICNAME. Normally, GDB knows in advance which syscalls are valid for each OS, so you can use the GDB command-line completion facilities (see command completion) to list the available choices. You may also specify the system call numerically. A syscall’s number is the value passed to the OS’s syscall dispatcher to identify the requested service. % gdb broken This only starts the debugger; it does not start running the program in the debugger. Look at the source code and set a breakpoint at line 43 (gdb) b 43 which is double seriesValue = ComputeSeriesValue(x, n); Now, we start to run the program in the debugger. GDB: The GNU Project Debugger bugs GDB Maintainers contributing current git documentation download home irc links mailing lists news schedule song wiki.
In gdb 7, support for extending gdb with Python wasadded. When CPython is built you will notice a python-gdb.py
file in theroot directory of your checkout. Read the module docstring for details on howto use the file to enhance gdb for easier debugging of a CPython process.
To activate support, you must add the directory containing python-gdb.py
to GDB’s “auto-load-safe-path”. Put this in your ~/.gdbinit
file:
You can also add multiple paths, separated by :
.
Welcome To Guide Dogs For The Blind | Guide Dogs For The Blind
This is what a backtrace looks like (truncated) when this extension isenabled:
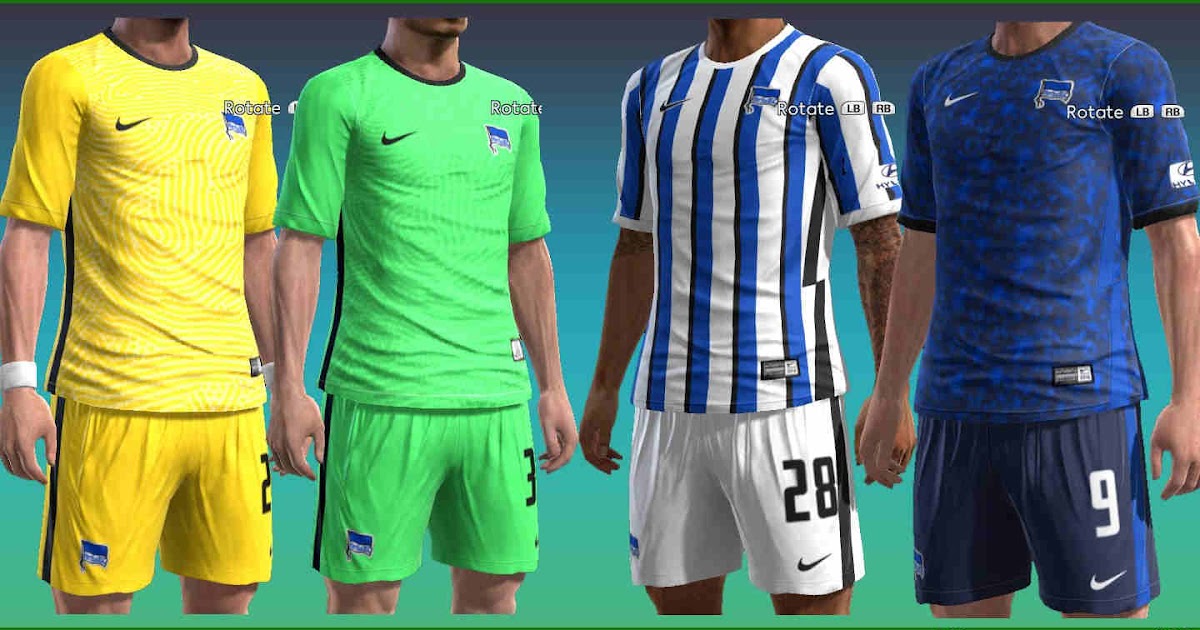
(Notice how the dictionary argument to PyDict_GetItemString
is displayedas its repr()
, rather than an opaque PyObject*
pointer.)
The extension works by supplying a custom printing routine for values of typePyObject*
. If you need to access lower-level details of an object, thencast the value to a pointer of the appropriate type. For example:
The pretty-printers try to closely match the repr()
implementation of theunderlying implementation of Python, and thus vary somewhat between Python 2and Python 3.
An area that can be confusing is that the custom printer for some types look alot like gdb’s built-in printer for standard types. For example, thepretty-printer for a Python 3 int
gives a repr()
that is notdistinguishable from a printing of a regular machine-level integer:
A similar confusion can arise with the str
type, where the output looks alot like gdb’s built-in printer for char*
:
The pretty-printer for str
instances defaults to using single-quotes (asdoes Python’s repr
for strings) whereas the standard printer for char*
values uses double-quotes and contains a hexadecimal address:
Here’s how to see the implementation details of a str
instance (for Python3, where a str
is a PyUnicodeObject*
):
As well as adding pretty-printing support for PyObject*
,the extension adds a number of commands to gdb:
py-list
List the Python source code (if any) for the current frame in the selectedthread. The current line is marked with a “>”:
Use py-listSTART
to list at a different line number within the pythonsource, and py-listSTART,END
to list a specific range of lines withinthe python source.
py-up
and py-down
The py-up
and py-down
commands are analogous to gdb’s regular up
and down
commands, but try to move at the level of CPython frames, ratherthan C frames.
gdb is not always able to read the relevant frame information, depending onthe optimization level with which CPython was compiled. Internally, thecommands look for C frames that are executing PyEval_EvalFrameEx
(whichimplements the core bytecode interpreter loop within CPython) and look upthe value of the related PyFrameObject*
.
They emit the frame number (at the C level) within the thread.
For example:
so we’re at the top of the python stack. Going back down:
and we’re at the bottom of the python stack.
py-bt
Further Information
The py-bt
command attempts to display a Python-level backtrace of thecurrent thread.
For example:
The frame numbers correspond to those displayed by gdb’s standardbacktrace
command.
Gdb Online
py-print
The py-print
command looks up a Python name and tries to print it.It looks in locals within the current thread, then globals, then finallybuiltins:
py-locals
The py-locals
command looks up all Python locals within the currentPython frame in the selected thread, and prints their representations:
You can of course use other gdb commands. For example, the frame
commandtakes you directly to a particular frame within the selected thread.We can use it to go a specific frame shown by py-bt
like this:
What Is The Difference Between LLDB And GDB - Pediaa.Com
The infothreads
command will give you a list of the threads within theprocess, and you can use the thread
command to select a different one:
You can use threadapplyallCOMMAND
or (taaCOMMAND
for short) to runa command on all threads. You can use this with py-bt
to see what everythread is doing at the Python level:
Tutorial: Debugging Embedded Devices Using GDB - Chris Simmonds, 2net Ltd
Note
RMS's Gdb Tutorial: How Do I Use Breakpoints?
This is only available for Python 2.7, 3.2 and higher.
gdb 6 and earlier¶
The file at Misc/gdbinit
contains a gdb configuration file which providesextra commands when working with a CPython process. To register these commandspermanently, either copy the commands to your personal gdb configuration fileor symlink ~/.gdbinit
to Misc/gdbinit
. To use these commands froma single gdb session without registering them, type sourceMisc/gdbinit
from your gdb session.
Updating auto-load-safe-path to allow test_gdb to run¶
test_gdb
attempts to automatically load additional Python specifichooks into gdb in order to test them. Unfortunately, the command lineoptions it uses to do this aren’t always supported correctly.
If test_gdb
is being skipped with an “auto-loading has been declined”message, then it is necessary to identify any Python build directories asauto-load safe. One way to achieve this is to add a line like the followingto ~/.gdbinit
(edit the specific list of paths as appropriate):